Couchbase Capella is made for developers, to amplify your skills and increase productivity. Use Capella for free today
SDKs by language
Couchbase provides SDKs for many programming languages. Choose your favorite, and we’ll show you how to get started, build applications, and get help.
Start building with Java
-
Getting started with Java
Includes synchronous APIs, plus reactive and asynchronous equivalents to maximize flexibility and performance.
-
Build applications
Program interactions with Couchbase via the Data, Query, and Search Services. Use the new collections feature.
-
Get help
Share knowledge, ask questions, and connect with fellow coders in the Java forum.
Java integrations
The perfect match of Couchbase and Spring Data integration. Manage data and boost your development productivity with this essential integration for Couchbase Capella or Server and the familiar ease of Spring Data.
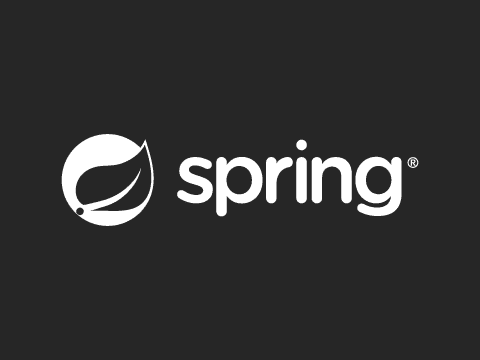
This official plugin provides integrated support for Couchbase within the entire JetBrains ecosystems (RubyMine, PyCharm, Rider, etc.), making it easier to interact with your Couchbase databases directly from your IDE.
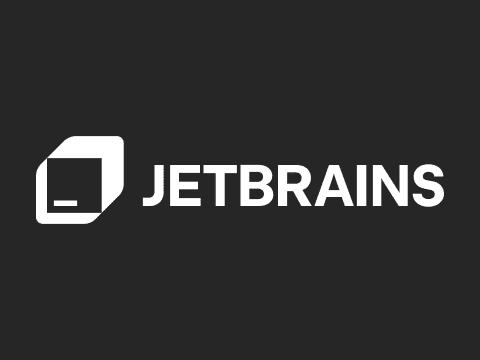
Seamlessly integrate Couchbase with Quarkus for high-performance applications, featuring GraalVM native image generation for lightning-fast, cloud-native execution.
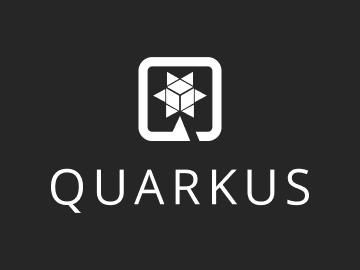
Start building with Scala
-
Getting started with Scala
Includes synchronous APIs, plus reactive and asynchronous equivalents to maximize flexibility and performance.
-
Build applications
Learn to program with Couchbase Server using our Travel Sample App and data, query, and search services.
-
Get help
Forums to learn and share ideas on OOP, functional programming, and advanced concepts.
Start building with .NET
-
Getting started with .NET
Interact with Couchbase from .NET using C# and other .NET languages. Asynchronous API is based on the TAP pattern.
-
Build applications
Try Couchbase .NET SDK and Travel Sample App. Launch with Docker Compose, explore backend, data model, and REST API.
-
Get help
Join the developer forum discussing Microsoft's framework and tools.
Start building with C
-
Getting started with C
The Couchbase C SDK enables you to interact with a Couchbase Server cluster using the C language.
-
Build applications
Discover how to use Couchbase Server's C SDK using our Travel Sample App.
-
Get help
Discuss and solve programming problems, share code, and connect with other C enthusiasts.
Start building with Node.js
-
Getting started with Node.js
Interact with a Couchbase Server or Capella cluster from the Node.js runtime, using TypeScript or JavaScript.
-
Build applications
Use our sample app code to start coding with the Couchbase Node.js SDK.
-
Get help
A forum for developers to discuss server-side JavaScript and solve issues.
Node.js integrations
Power your website with seamless Couchbase Capella integration on Netlify. Optimize your web applications effortlessly and increase productivity. Boost performance and scalability with the combination of Netlify and Couchbase.
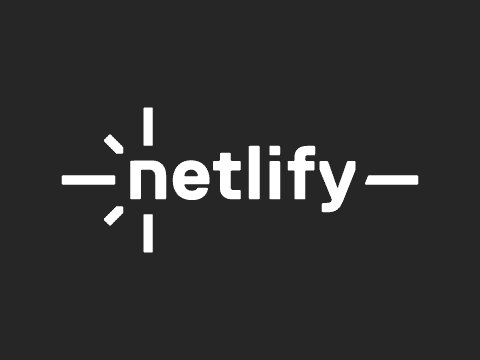
The official Couchbase extension for Visual Studio Code, offering a seamless integration with the powerful editor. Enhanced productivity and convenience, empowering Couchbase Capella and Server users to work within the familiar environment of VS Code.
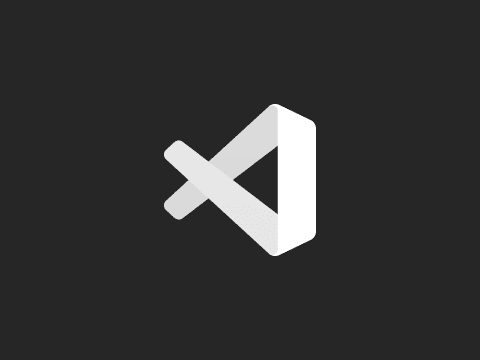
Ottoman is an ODM built for Couchbase and Node.js. The official Couchbase extension for Visual Studio Code, offering a seamless integration with the powerful editor. Enhanced productivity and convenience, empowering Couchbase Capella and Server users to work within the familiar environment of VS Code.
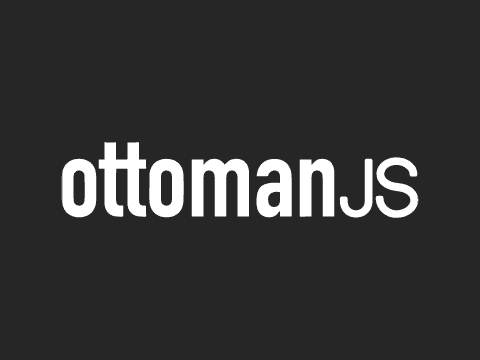
Start building with PHP
-
Getting started with PHP
Connect to a Couchbase cluster using PHP. This native PHP extension uses the Couchbase C++ library.
-
Build applications
Start coding with the Couchbase PHP SDK using our Travel Sample App and sample data set.
-
Get help
Connect with other PHP developers, share code, and learn the ins and outs of this popular language.
Start building with Python
-
Getting started with Python
Python applications can access a Couchbase cluster using traditional synchronous API, Twisted, and asyncio.
-
Build applications
Use the Python SDK for Couchbase to interact with data, query, and search services using our Travel Sample App.
-
Get help
Discuss Python programming language, share insights, and get help from the community.
Start building with GO
-
Getting started with Go
Build Go applications that interact with Couchbase for data storage and retrieval.
-
Build applications
Build an interactive application with Couchbase using the Go SDK and the Travel Sample App.
-
Get help
Join the growing community of developers building systems with Go and share your expertise.
Start building with Kotlin
-
Getting started with Kotlin
A Kotlin application running on the JVM can use the Couchbase Kotlin SDK to access a Couchbase cluster.
-
Build applications
Connect to a Couchbase Capella or Couchbase Server cluster using Kotlin SDK.
-
Get help
Join the community of Kotlin enthusiasts and discuss everything related to Kotlin and Couchbase.
Start building with Ruby
-
Getting started with Ruby
The Ruby SDK includes native Ruby extensions for Couchbase’s binary protocols.
-
Build applications
Program interactions with Couchbase Server using our Travel Sample App and data, query, and search services.
-
Get help
Join the forum for Ruby developers, share code, and get help from experienced programmers.
Browse by tool type
Couchbase offers a range of tools that are designed to help users manage and work with Couchbase Capella and Couchbase Server more effectively. These tools include the Couchbase Shell, SDK Doctor, big data connectors, and SDK extension libraries.
-
Capella Playground
Capella Playground is a web-based interface for exploring Couchbase Server features. You can use it to create and interact with sample data, test queries, and experiment with full-text search. It generates code snippets in multiple programming languages so you can learn, test, and prototype.
-
Couchbase Shell
Couchbase Shell is a command-line interface for managing Couchbase Server that allows admins to perform tasks like cluster config, node monitoring, and data backup and restore. It supports scripting languages, automates common tasks, and is useful for tasks not easily accessible through the web UI.
-
Big data connectors
Couchbase connectors for big data integrations include:
• Kafka – for real-time data processing and analytics
• Elasticsearch – for full-text search and indexing of data stored in Couchbase
• Tableau – for visual analysis and reporting on Couchbase data
• Spark – for efficient processing of large data sets -
SDK Doctor
Couchbase SDK Doctor is a command-line tool for Couchbase Server SDKs that diagnoses network connectivity issues, verifies configurations, and tests for common errors. It runs on Windows, macOS, and Linux to help developers and admins troubleshoot and ensure proper SDK usage.
-
SDK extension libraries
Couchbase SDK extension libraries are add-ons that provide additional functionality to the Couchbase SDKs. Examples include Distributed ACID Transactions, Field Level Encryption, Response Time Observability, and Spring Data for Couchbase.